Learn Concurrent Programming with Go: A Comprehensive Guide
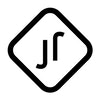
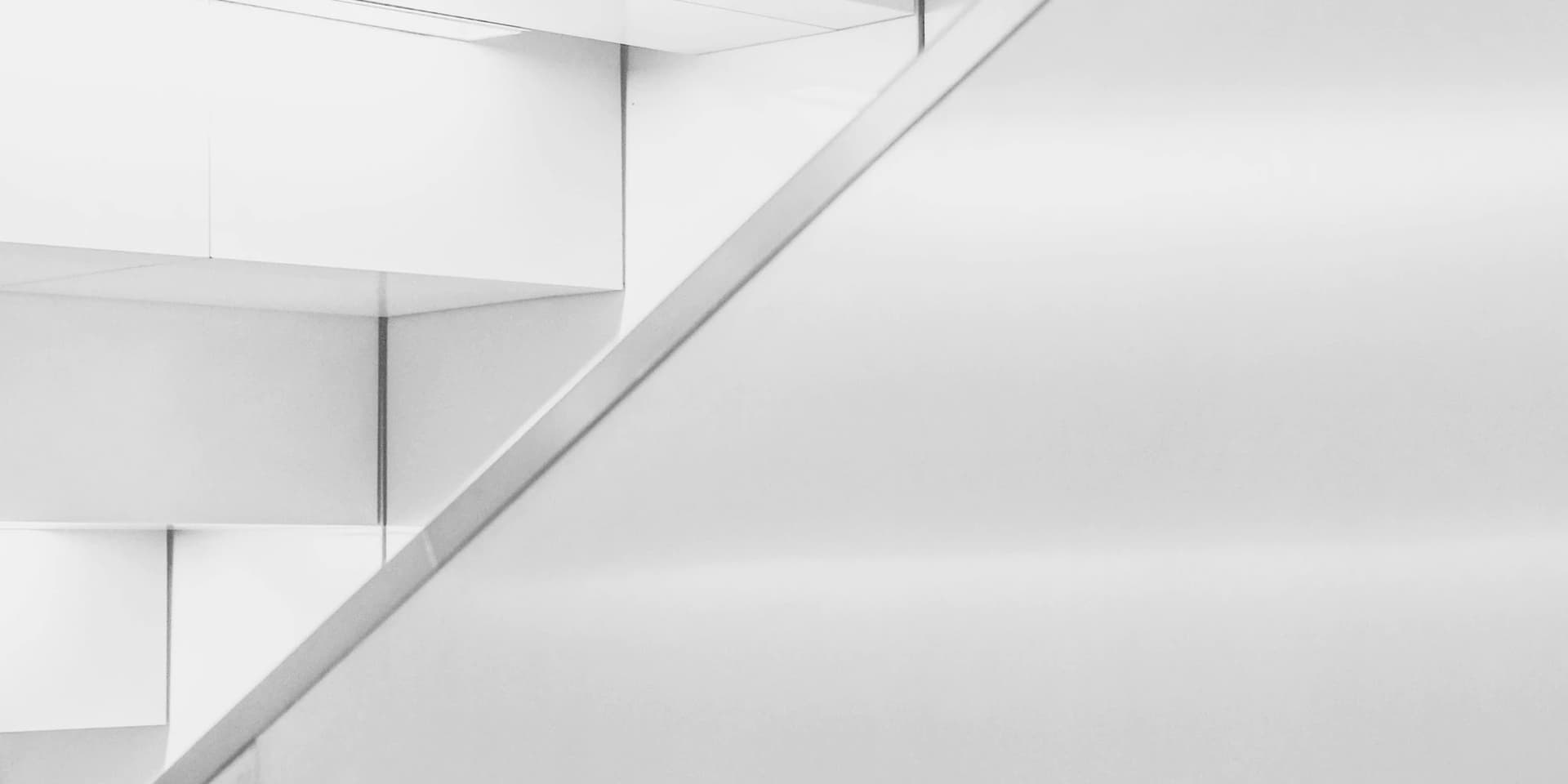
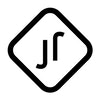
Learn Concurrent Programming with Go: A Comprehensive Guide
ā
Introduction
Concurrency is a fundamental concept in modern programming that allows multiple tasks to execute and interact simultaneously, improving performance and responsiveness. One language that excels in concurrency is Go. In this article, we will explore the principles and techniques of concurrent programming in Go, providing you with a comprehensive guide to harnessing the power of Go's concurrency features.
Understanding Concurrency and Parallelism
Before delving into the specifics of concurrent programming in Go, let's first clarify the difference between concurrency and parallelism. Concurrency refers to a programming language's ability to handle multiple tasks simultaneously. It involves dividing a program into smaller, independent units of execution, known as goroutines in Go, which can run concurrently. On the other hand, parallelism involves executing multiple tasks simultaneously using multiple CPUs.
To better understand concurrency, imagine multiple cars traveling on two lanes. Sometimes, the cars overtake each other, and sometimes they stop and let others pass by. This dynamic interaction between cars is analogous to the execution of concurrent tasks in a program. In contrast, parallelism can be visualized as cars traveling on separate roads, each isolated and independent from the others.
In Go, goroutines provide the means to achieve concurrency. Goroutines are lightweight threads of execution managed by the Go runtime. They allow you to execute functions independently and concurrently, enabling efficient utilization of system resources.
Goroutines: Lightweight Concurrency in Go
One of the key features of Go is its support for goroutines, which are lightweight threads of execution that can run concurrently. Goroutines make it easy to implement concurrent tasks in Go, allowing you to execute functions independently and concurrently.
To create a goroutine, you simply prefix a function call with the go
keyword. Here's an example:
package main
import (
"fmt"
"time"
)
func main() {
go sayHello()
time.Sleep(1 \* time.Second)
}
func sayHello() {
fmt.Println("Hello, World!")
}
In this example, the sayHello()
function is executed as a goroutine. The main()
function starts the goroutine with the go
keyword and then waits for 1 second using time.Sleep()
before exiting. Without the sleep statement, the program would exit before the sayHello()
goroutine has a chance to complete its execution.
By utilizing goroutines, you can achieve concurrent execution of tasks, improving the responsiveness and performance of your Go programs.
Synchronization with WaitGroups
In concurrent programming, it is often necessary to wait for multiple goroutines to finish their execution before proceeding. Go provides a synchronization mechanism called WaitGroups to achieve this.
A WaitGroup is a simple construct that allows you to wait for a collection of goroutines to complete their execution. It works by maintaining an internal counter that is incremented when a goroutine starts and decremented when it finishes. The Wait()
method blocks the execution of the current goroutine until the internal counter becomes zero.
Here's an example that demonstrates the use of WaitGroups:
package main
import (
"fmt"
"sync"
)
func main() {
var wg sync.WaitGroup
wg.Add(2)
go printMessage("Hello", &wg)
go printMessage("World", &wg)
wg.Wait()
}
func printMessage(message string, wg \*sync.WaitGroup) {
defer wg.Done()
fmt.Println(message)
}
In this example, the main()
function creates a WaitGroup and adds two goroutines to it. Each goroutine calls the printMessage()
function, which simply prints a message. The Done()
method is deferred to ensure that the internal counter is decremented when the goroutine finishes.
By utilizing WaitGroups, you can ensure that all necessary goroutines have completed their execution before continuing with the rest of your program.
Communication with Channels
In concurrent programming, communication and synchronization between goroutines are essential. Go provides a powerful mechanism called channels to facilitate bidirectional communication between goroutines.
A channel is a typed conduit through which you can send and receive values between goroutines. It ensures safe and synchronized communication by enforcing synchronization points, where the sender blocks until the receiver is ready to receive the value.
Channels in Go can be either unbuffered or buffered. Unbuffered channels require both the sender and receiver to be present for a successful operation. It requires a goroutine to read the data; otherwise, it will lead to a deadlock. Buffered channels, on the other hand, have the capacity to store values for future processing. The sender is not blocked until the channel becomes full, and it doesn't necessarily need a reader to complete the synchronization.
To declare a channel in Go, you can use the following syntax:
ch :\= make(chan Type)
You can also declare channels based on their directions. Bidirectional channels allow both sending and receiving, send-only channels only allow sending, and receive-only channels only allow receiving.
Channels are an effective way to send and receive notifications and can be used to implement various communication patterns, such as pipelining, worker pools, and message passing.
Error Handling in Concurrent Programs
Error handling in concurrent programs can be challenging due to the non-deterministic nature of concurrent execution. When multiple goroutines are executing concurrently, it's crucial to handle errors properly to ensure the correctness and reliability of your program.
In Go, one approach to error handling in concurrent programs is to use the error
type and the select
statement. The select
statement allows you to wait for multiple channel operations simultaneously. By combining the select
statement with the error
type, you can propagate errors from goroutines to the main program.
Here's an example that demonstrates error handling in concurrent programs:
package main
import (
"errors"
"fmt"
"time"
)
func main() {
errCh :\= make(chan error)
go performTask(errCh)
select {
case err :\= <\-errCh:
if err != nil {
fmt.Println("Error:", err)
}
case <\-time.After(5 \* time.Second):
fmt.Println("Timed out")
}
}
func performTask(errCh chan error) {
time.Sleep(3 \* time.Second)
errCh <\- errors.New("Something went wrong")
}
In this example, the performTask()
function simulates a task that takes 3 seconds to complete. If an error occurs during the execution of the task, it is sent through the errCh
channel. The main()
function uses the select
statement to wait for either an error or a timeout. If an error is received, it is printed to the console.
By combining error handling techniques with concurrent programming constructs, you can effectively handle errors in your concurrent Go programs.
Advanced Topics in Concurrent Programming
While the previous sections covered the fundamentals of concurrent programming in Go, there are several advanced topics worth exploring to further enhance your concurrency skills.
Atomic Operations
In concurrent programming, atomic operations are essential for ensuring the correctness of shared data accessed by multiple goroutines. Go provides the atomic
package, which offers atomic operations for common data types such as integers and booleans. By using atomic operations, you can avoid race conditions and guarantee the integrity of shared data.
Here's an example that demonstrates atomic operations in Go:
package main
import (
"fmt"
"sync"
"sync/atomic"
)
func main() {
var counter int64
var wg sync.WaitGroup
wg.Add(10)
for i :\= 0; i < 10; i++ {
go incrementCounter(&counter, &wg)
}
wg.Wait()
fmt.Println("Counter:", counter)
}
func incrementCounter(counter \*int64, wg \*sync.WaitGroup) {
defer wg.Done()
atomic.AddInt64(counter, 1)
}
In this example, multiple goroutines increment a counter using the atomic.AddInt64()
function from the atomic
package. The atomic
package provides atomic operations for various data types, allowing you to safely manipulate shared data in concurrent programs.
Mutexes and RWMutexes
Mutexes and RWMutexes are synchronization primitives in Go that provide exclusive access and shared access to a resource, respectively. They are useful for protecting shared data from concurrent modifications and ensuring data consistency.
A Mutex allows only one goroutine to access a resource at a time, while an RWMutex allows multiple goroutines to read the resource simultaneously. However, when a goroutine wants to write to the resource, it must acquire an exclusive lock, preventing other goroutines from reading or writing.
Here's an example that demonstrates the use of Mutex and RWMutex in Go:
package main
import (
"fmt"
"sync"
"time"
)
func main() {
var counter int
var wg sync.WaitGroup
var mutex sync.Mutex
wg.Add(10)
for i :\= 0; i < 5; i++ {
go incrementCounter(&counter, &mutex, &wg)
go decrementCounter(&counter, &mutex, &wg)
}
wg.Wait()
fmt.Println("Counter:", counter)
}
func incrementCounter(counter \*int, mutex \*sync.Mutex, wg \*sync.WaitGroup) {
defer wg.Done()
mutex.Lock()
\*counter++
mutex.Unlock()
}
func decrementCounter(counter \*int, mutex \*sync.Mutex, wg \*sync.WaitGroup) {
defer wg.Done()
mutex.Lock()
\*counter\--
mutex.Unlock()
}
In this example, multiple goroutines increment and decrement a counter using a Mutex to ensure exclusive access to the counter
variable. The Lock()
and Unlock()
methods of the Mutex are used to acquire and release the lock, respectively.
Context Package
The Context package in Go provides a way to manage the lifecycle of goroutines and propagate cancellation signals across goroutines. It is particularly useful in concurrent programs that involve long-running tasks or multiple levels of goroutine hierarchy.
By using the Context package, you can avoid goroutine leaks and gracefully handle the cancellation of goroutines. It allows you to pass a Context object to your goroutines, which can be used to check for cancellation signals and propagate them to child goroutines.
Here's an example that demonstrates the use of the Context package in Go:
package main
import (
"context"
"fmt"
"time"
)
func main() {
ctx :\= context.Background()
ctx, cancel :\= context.WithCancel(ctx)
go performTask(ctx)
time.Sleep(2 \* time.Second)
cancel()
time.Sleep(1 \* time.Second)
fmt.Println("Main goroutine exited")
}
func performTask(ctx context.Context) {
for {
select {
case <\-ctx.Done():
fmt.Println("Task canceled")
return
default:
fmt.Println("Performing task...")
time.Sleep(500 \* time.Millisecond)
}
}
}
In this example, the performTask()
function performs a long-running task inside a goroutine. The Context object is passed to the goroutine, allowing it to check for cancellation signals using the Done()
method. When the main goroutine calls the cancel()
function, the performTask()
goroutine receives a cancellation signal and exits gracefully.
By utilizing the Context package, you can effectively manage the lifecycle of goroutines and handle cancellation signals in concurrent programs.
Conclusion
In conclusion, concurrent programming in Go provides a powerful way to improve the performance and responsiveness of your applications. By utilizing goroutines, channels, synchronization primitives, and advanced concurrency techniques, you can harness the full potential of Go's concurrency features.
In this article, we explored the fundamentals of concurrent programming in Go, including goroutines, synchronization with WaitGroups, communication with channels, error handling, and advanced topics such as atomic operations, mutexes, RWMutexes, and the Context package.
By mastering concurrent programming in Go, you can develop highly efficient and scalable applications that take full advantage of modern multi-processor hardware. So go ahead, dive into the world of concurrent programming with Go, and unlock the full potential of your software. Happy coding!
Additional Information:
- It is important to note that concurrent programming requires careful consideration and proper design to handle potential issues such as deadlocks, race conditions, and resource contention. It is recommended to thoroughly test and validate your concurrent programs to ensure correctness and reliability.
- The Go programming language provides a rich set of libraries and tools for concurrent programming, including the
sync
package, which offers synchronization primitives like Mutexes, RWMutexes, and WaitGroups. Additionally, the standard library provides theatomic
package for atomic operations and thecontext
package for managing the lifecycle of goroutines. - When designing concurrent programs, it is crucial to follow best practices, such as minimizing shared mutable state, avoiding unnecessary synchronization, and using appropriate synchronization primitives for different scenarios.
- It is also worth exploring advanced concurrency patterns and techniques, such as fan-out/fan-in, worker pools, and message passing, to further optimize and scale your concurrent programs.